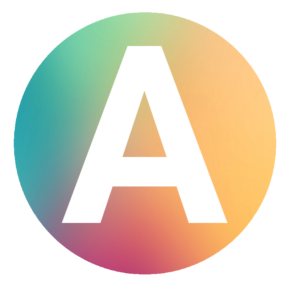 Add IVCProof to the existing folding schemes (Nova,HyperNova,ProtoGalaxy) (#167)
* Add IVCProof to the existing folding schemes (Nova,HyperNova,ProtoGalaxy)
* Implement `from_ivc_proof` for the FoldingSchemes trait (and Nova,
HyperNova, ProtoGalaxy), so that the FoldingScheme IVC's instance can be
constructed from the given parameters and the last IVCProof, which
allows to sent the IVCProof between different parties, so that they can
continue iterating the IVC from the received IVCProof. Also the
serializers allow for the IVCProof to be sent to a verifier that can
deserialize it and verify it.
This allows to remove the logic from the file
[folding/nova/serialize.rs](https://github.com/privacy-scaling-explorations/sonobe/blob/f1d82418ba047cf90805f2d0505370246df24d68/folding-schemes/src/folding/nova/serialize.rs)
and
[folding/hypernova/serialize.rs](https://github.com/privacy-scaling-explorations/sonobe/blob/f1d82418ba047cf90805f2d0505370246df24d68/folding-schemes/src/folding/hypernova/serialize.rs)
(removing the whole files), which is now covered by the `IVCProof`
generated serializers (generated by macro instead of handwritten), and
the test that the file contained is now abstracted and applied to all
the 3 existing folding schemes (Nova, HyperNova, ProtoGalaxy) at the
folding/mod.rs file.
* update Nova VerifierParams serializers to avoid serializing the R1CS to save big part of the old serialized size
* rm .instances() since it's not needed
* add nova params serialization to nova's ivc test to ensure that IVC verification works with deserialized data
* Add unified FS::ProverParam & VerifierParam serialization & deserialization (for all Nova, HyperNova and ProtoGalaxy), without serializing the R1CS/CCS and thus saving substantial serialized bytes space.
* rm CanonicalDeserialize warnings msgs for VerifierParams 1 month ago 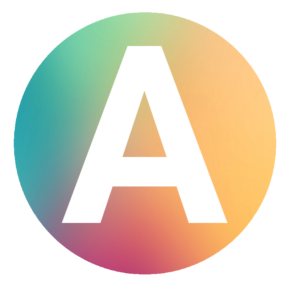 Add IVCProof to the existing folding schemes (Nova,HyperNova,ProtoGalaxy) (#167)
* Add IVCProof to the existing folding schemes (Nova,HyperNova,ProtoGalaxy)
* Implement `from_ivc_proof` for the FoldingSchemes trait (and Nova,
HyperNova, ProtoGalaxy), so that the FoldingScheme IVC's instance can be
constructed from the given parameters and the last IVCProof, which
allows to sent the IVCProof between different parties, so that they can
continue iterating the IVC from the received IVCProof. Also the
serializers allow for the IVCProof to be sent to a verifier that can
deserialize it and verify it.
This allows to remove the logic from the file
[folding/nova/serialize.rs](https://github.com/privacy-scaling-explorations/sonobe/blob/f1d82418ba047cf90805f2d0505370246df24d68/folding-schemes/src/folding/nova/serialize.rs)
and
[folding/hypernova/serialize.rs](https://github.com/privacy-scaling-explorations/sonobe/blob/f1d82418ba047cf90805f2d0505370246df24d68/folding-schemes/src/folding/hypernova/serialize.rs)
(removing the whole files), which is now covered by the `IVCProof`
generated serializers (generated by macro instead of handwritten), and
the test that the file contained is now abstracted and applied to all
the 3 existing folding schemes (Nova, HyperNova, ProtoGalaxy) at the
folding/mod.rs file.
* update Nova VerifierParams serializers to avoid serializing the R1CS to save big part of the old serialized size
* rm .instances() since it's not needed
* add nova params serialization to nova's ivc test to ensure that IVC verification works with deserialized data
* Add unified FS::ProverParam & VerifierParam serialization & deserialization (for all Nova, HyperNova and ProtoGalaxy), without serializing the R1CS/CCS and thus saving substantial serialized bytes space.
* rm CanonicalDeserialize warnings msgs for VerifierParams 1 month ago |
|
#![allow(non_snake_case)]
#![allow(non_upper_case_globals)]
#![allow(non_camel_case_types)]
#![allow(clippy::upper_case_acronyms)]
use ark_crypto_primitives::crh::{
sha256::{
constraints::{Sha256Gadget, UnitVar},
Sha256,
},
CRHScheme, CRHSchemeGadget,
};
use ark_ff::{BigInteger, PrimeField, ToConstraintField};
use ark_r1cs_std::{fields::fp::FpVar, ToBytesGadget, ToConstraintFieldGadget};
use ark_relations::r1cs::{ConstraintSystemRef, SynthesisError};
use core::marker::PhantomData;
use std::time::Instant;
use ark_bn254::{constraints::GVar, Bn254, Fr, G1Projective as Projective};
use ark_grumpkin::{constraints::GVar as GVar2, Projective as Projective2};
use folding_schemes::commitment::{kzg::KZG, pedersen::Pedersen};
use folding_schemes::folding::nova::{Nova, PreprocessorParam};
use folding_schemes::frontend::FCircuit;
use folding_schemes::transcript::poseidon::poseidon_canonical_config;
use folding_schemes::{Error, FoldingScheme};
/// This is the circuit that we want to fold, it implements the FCircuit trait.
/// The parameter z_i denotes the current state, and z_{i+1} denotes the next state which we get by
/// applying the step.
/// In this example we set z_i and z_{i+1} to be a single value, but the trait is made to support
/// arrays, so our state could be an array with different values.
#[derive(Clone, Copy, Debug)]
pub struct Sha256FCircuit<F: PrimeField> {
_f: PhantomData<F>,
}
impl<F: PrimeField> FCircuit<F> for Sha256FCircuit<F> {
type Params = ();
fn new(_params: Self::Params) -> Result<Self, Error> {
Ok(Self { _f: PhantomData })
}
fn state_len(&self) -> usize {
1
}
fn external_inputs_len(&self) -> usize {
0
}
/// computes the next state values in place, assigning z_{i+1} into z_i, and computing the new
/// z_{i+1}
fn step_native(
&self,
_i: usize,
z_i: Vec<F>,
_external_inputs: Vec<F>,
) -> Result<Vec<F>, Error> {
let out_bytes = Sha256::evaluate(&(), z_i[0].into_bigint().to_bytes_le()).unwrap();
let out: Vec<F> = out_bytes.to_field_elements().unwrap();
Ok(vec![out[0]])
}
/// generates the constraints for the step of F for the given z_i
fn generate_step_constraints(
&self,
_cs: ConstraintSystemRef<F>,
_i: usize,
z_i: Vec<FpVar<F>>,
_external_inputs: Vec<FpVar<F>>,
) -> Result<Vec<FpVar<F>>, SynthesisError> {
let unit_var = UnitVar::default();
let out_bytes = Sha256Gadget::evaluate(&unit_var, &z_i[0].to_bytes()?)?;
let out = out_bytes.0.to_constraint_field()?;
Ok(vec![out[0].clone()])
}
}
/// cargo test --example sha256
#[cfg(test)]
pub mod tests {
use super::*;
use ark_r1cs_std::{alloc::AllocVar, R1CSVar};
use ark_relations::r1cs::ConstraintSystem;
// test to check that the Sha256FCircuit computes the same values inside and outside the circuit
#[test]
fn test_f_circuit() {
let cs = ConstraintSystem::<Fr>::new_ref();
let circuit = Sha256FCircuit::<Fr>::new(()).unwrap();
let z_i = vec![Fr::from(1_u32)];
let z_i1 = circuit.step_native(0, z_i.clone(), vec![]).unwrap();
let z_iVar = Vec::<FpVar<Fr>>::new_witness(cs.clone(), || Ok(z_i)).unwrap();
let computed_z_i1Var = circuit
.generate_step_constraints(cs.clone(), 0, z_iVar.clone(), vec![])
.unwrap();
assert_eq!(computed_z_i1Var.value().unwrap(), z_i1);
}
}
/// cargo run --release --example sha256
fn main() {
let num_steps = 10;
let initial_state = vec![Fr::from(1_u32)];
let F_circuit = Sha256FCircuit::<Fr>::new(()).unwrap();
/// The idea here is that eventually we could replace the next line chunk that defines the
/// `type N = Nova<...>` by using another folding scheme that fulfills the `FoldingScheme`
/// trait, and the rest of our code would be working without needing to be updated.
type N = Nova<
Projective,
GVar,
Projective2,
GVar2,
Sha256FCircuit<Fr>,
KZG<'static, Bn254>,
Pedersen<Projective2>,
false,
>;
let poseidon_config = poseidon_canonical_config::<Fr>();
let mut rng = rand::rngs::OsRng;
println!("Prepare Nova ProverParams & VerifierParams");
let nova_preprocess_params = PreprocessorParam::new(poseidon_config, F_circuit);
let nova_params = N::preprocess(&mut rng, &nova_preprocess_params).unwrap();
println!("Initialize FoldingScheme");
let mut folding_scheme = N::init(&nova_params, F_circuit, initial_state.clone()).unwrap();
// compute a step of the IVC
for i in 0..num_steps {
let start = Instant::now();
folding_scheme.prove_step(rng, vec![], None).unwrap();
println!("Nova::prove_step {}: {:?}", i, start.elapsed());
}
println!("Run the Nova's IVC verifier");
let ivc_proof = folding_scheme.ivc_proof();
N::verify(
nova_params.1, // Nova's verifier params
ivc_proof,
)
.unwrap();
}
|